- Published on
How to Enable Audio Playback on Safari, Chrome, and iPhone (Cross-Platform) A Developer's Guide
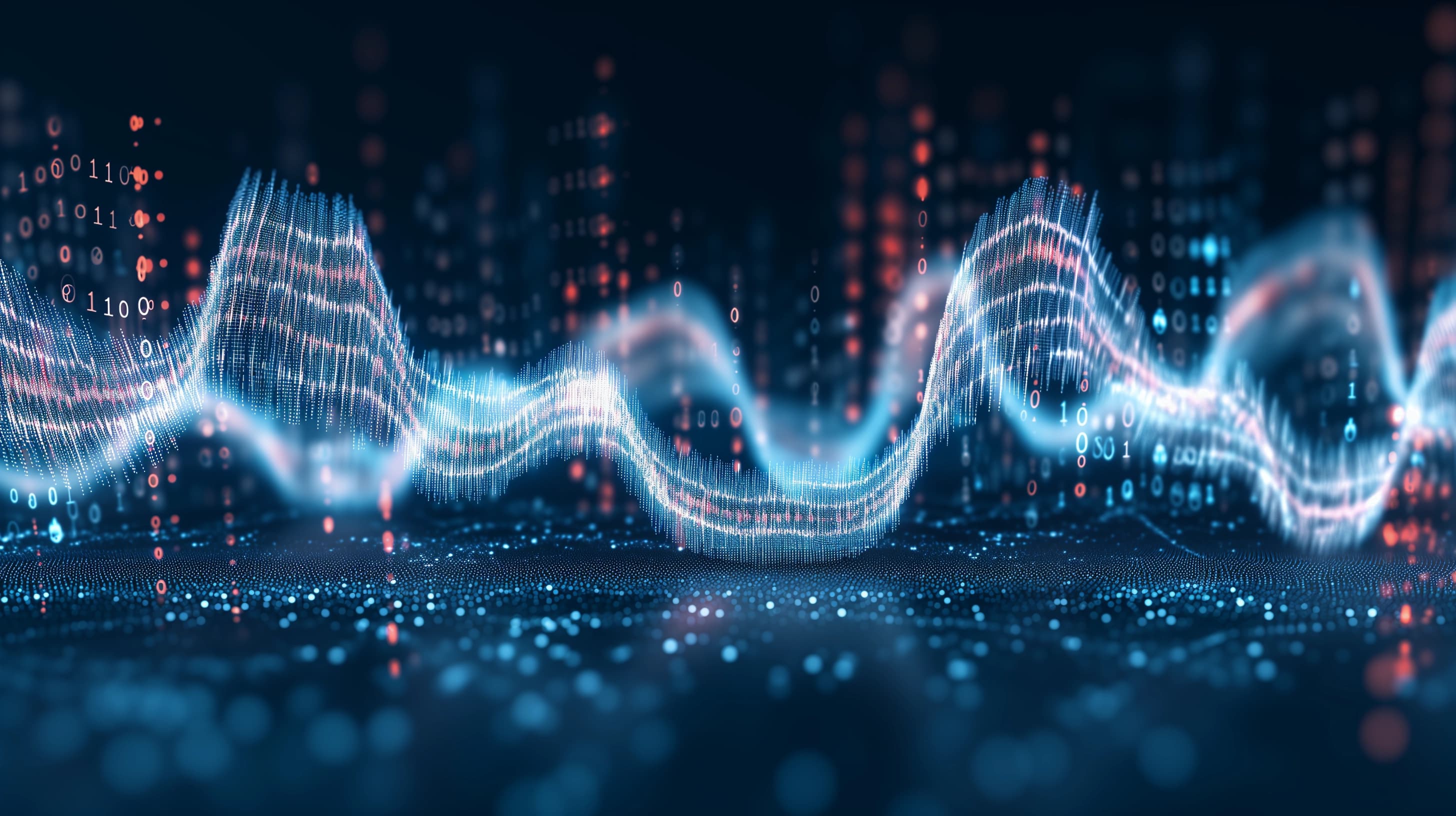
Cross-platform audio playback is a crucial feature for any web application, especially when media is at the heart of the user experience. However, developers often face hurdles when their perfectly fine-tuned local setups falter on different devices or browsers, particularly Safari. Here's how we tackled these challenges at FluentlyFlow, a language learning platform that leverages the power of music.
The Common Challenge: Safari Audio Playback
When developing FluentlyFlow, our tests on local development environments showed promising results—until we ventured into real-world testing across various devices, including iPhones and iPads. Safari, known for its stringent auto-play policies and unique handling of audio contexts, posed the most significant challenges.
Unlocking Audio Across Browsers
To address these issues and ensure that our users could experience uninterrupted audio on any device, we implemented a specific function to unlock audio playback capabilities across all browsers, including Safari. Here’s a closer look at the function and how it works:
JavaScript Function to Unlock Audio
function unlockAudio() {
// Initialize Audio Context
const audioContext = new (window.AudioContext || window.webkitAudioContext)()
const buffer = audioContext.createBuffer(1, 1, 22050)
const source = audioContext.createBufferSource()
source.buffer = buffer
source.connect(audioContext.destination)
source.start ? source.start(0) : source.noteOn(0)
// Check if audio is actually played
setTimeout(function () {
if (
source.playbackState === source.PLAYING_STATE ||
source.playbackState === source.FINISHED_STATE
) {
setIsUnlocked(true)
}
}, 0)
return () => audioContext.close()
}
How the Unlock Function Works
The unlockAudio function initiates an audio context and a buffer source node, playing a silent audio clip to 'unlock' the audio on the device. This method effectively circumvents the limitations imposed by browsers like Safari, which require user interaction to play audio. By running this function on a user's first interaction (like a tap), it enables audio playback throughout the session.
Implementing the Unlock Audio Function
To integrate this function into your web application:
- User Interaction Trigger: Attach the unlockAudio function to a user interaction event handler, such as a button click or page load, depending on your application’s flow.
- State Management: Utilize the setIsUnlocked state to manage and track whether the audio context has been successfully unlocked.
- Cleanup: Ensure to close the audio context when it's no longer needed to free up resources.
FluentlyFlow
Lessons Learned atOur journey at FluentlyFlow taught us that thorough testing across all potential user environments is crucial. By implementing the above function, we were able to enhance the user experience, ensuring that learners could engage with our language-through-music content without interruptions, irrespective of their device or browser.
Conclusion
Ensuring cross-platform compatibility for audio playback can be daunting, but with the right techniques and a bit of code, it's certainly achievable. Whether you're developing an educational platform like FluentlyFlow or any other media-rich web application, tackling audio playback issues head-on will lead to a smoother, more engaging user experience.
Embrace the challenge, and enhance your web applications by ensuring they speak clearly and loudly—on any platform.
For more insights and technical guides, keep exploring our blog at FluentlyFlow.co—where language learning meets music in perfect harmony.